728x90
320x100
클래스 Classes
타입스크립트에서는 파라미터들을 써주기만 하면 알아서 constructor함수를 만들어준다.
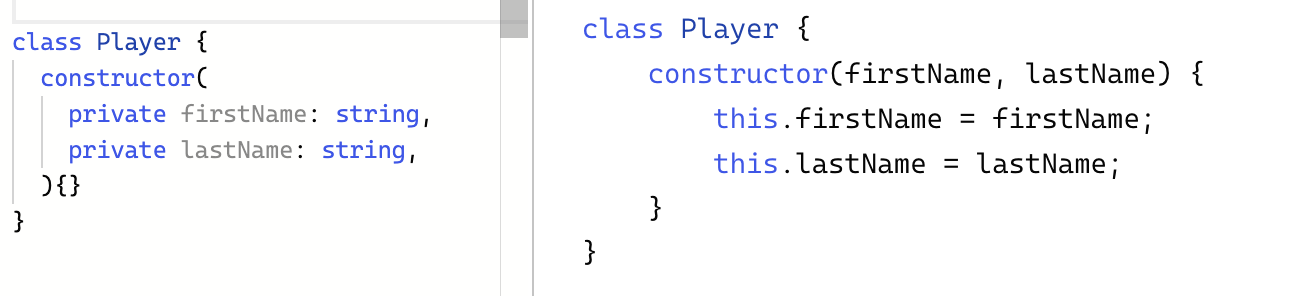
class Player {
constructor(
private firstName: string, //JS에서는 private 부분이 보이지 않는다.
private lastName: string,
public nickName: string,
){}
}
const player = new Player('firstName', 'last' , 'Tom');
player.firstName // -> class에서 private로 해주었기 때문에 오류가 나며 컴파일이 되지 않는다.
추상클래스, 추상메소드, private, protected, public
타입스크립드에서 제공하는 기능
추상클래스: 다른 클래스가 상속받을 수 있는 클래스. 직접 인스텐스가 불가하다
추상클래스는 타입스크립트에만 있는 기능이기 때문에 자바스크립트로 변환시 일반 class로 보인다. 그렇다면 왜 쓰는것일까?
-> 표준화된 프로퍼티와 메소드를 갖도록 해주는 청사진을 만들기 위함이다.
-> 인터페이스를 사용하면 컴파일시 Js로 바뀌지 않고 사라지기때문에 인터페이스를 사용한다.(추상 클래스를 다른 클래스들이 특정모양을 따르도록 하기 위한 용도로 쓰인다면 인터페이스를 쓰는것이 낫다.)
추상메소드: 추상메소드를 만드려면 메소드를 클래스 안에서 구현하지 않으면 된다.
추상메소드는 구현이 되어있지 않은(코드가 없는) 메소드이다. call cignature만 가지고 있는데, 함수의 이름과 argument를 안 받을 때도 있지만 argument를 받을 경우 argument의 이름과 타입 그리고 함수의 리턴타입을 정의하고 있다.
private: 자식클래스가 접근불가
protected: 자식클래스 접근가능, 외부접근 불가
public: 모두 접근가능
abstract class User{
constructor(
private firstName: string, // private로 되어있으면 자식클래스가 접근하지 못한다. 인스턴스화 할 수 없다.
protected lastName: string, // 자식클래스에서도 접근이 가능하게 하려면 protected를 쓰는것이 좋다.(외부에서는 사용불가)
public nickName: string,
){}
private getFullName(){ //-> 메소드도 private설정을 해줄 수 있다.
return `${this.firstName} ${this.lastName}`
}
}
class Player extends User{ //-> 추상클래스인 User을 상속받음 + 메소드인 getFullName도 상속받을 수 있다.
}
const user = new Player('firstName', 'last' , 'Tom');
user.getFullName() //-> 이런식으로 추상클래스에서 만든 메소드를 불러올 수 있다.
// 추상클래스에선 청사진만 제공한다. 메소드 구현은 자식클래스에서 구현.
abstract class User{
constructor(
protected fistName:string,
protected lastName:string
){}
abstract sayHi(name: string):string // 청사진만 제공. 메소드구현은 no
abstract fullName():string
}
class Player extends User{
fullName(){
return `${this.fullName} ${this.lastName}`
}
sayHi(){
return `hello ${name}. My name is ${this.fullName()}`
}
}
예제-해시맵으로 단어사전 만들기 (해싱알고리즘을 사용)
*해시맵이란?
해시(hash): 해시란 데이터를 다루는 기법 중에 하나로 검색과 저장이 아주 빠르게 진행된다. 데이터를 검색할 때 사용할 Key와 실제 데이터의 값(Value)이 한 쌍으로 존재하고, Key값이 배열의 인덱스로 변환되기 때문에 검색과 저장의 평균적인 시간 복잡도가 O(1)에 수렴하게 된다. 즉 데이터를 효율적으로 관리하기 위해, 임의의 길이 데이터를 고정된 길이의 데이터로 매핑하는 것
해시 함수를 구현하여 데이터 값을 해시 값으로 매핑한다.
맵 (map): 키(Key)와 값(Value) 두 쌍으로 데이터를 보관하는 자료구조
type Words = {
[key:string]: String //[key:string] 이런 형식은 object의 type을 선언해야할때 쓸 수 있다. 이 object는 제한된 양의 property만을 가질 수 있고, property에 대해서 미리 알진 못하지만 타입만 알고 있을때 쓰면 된다.
}
class Dict{
private words: Words //words를 initializer없이 선언해주고
constructor(){ //constructor에서 수동으로 초기화시킴
this.words = {}
}
add(word:Word){ //클래스를 type으로 쓰기 가능
if(this.words[word.term] === undefined){
this.words[word.term] = word.def;
}
}
def(term:string){
return this.words[term]
}
del(term:string){
delete this.words[term];
}
}
class Word {
constructor(
public term: string,
public def: string
){}
}
const tomato = new Word('tomato', '토마토');
const dict = new Dict()
dict.add(tomato);
dict.def('tomato')
728x90
320x100
'front-end > TypeScript' 카테고리의 다른 글
[TS]08 제네릭+다형성+클래스+인터페이스 (0) | 2022.05.17 |
---|---|
[TS]07 type과 interface (0) | 2022.05.16 |
[TS]05 다형성 / 제네릭 (0) | 2022.05.11 |
[TS]04 call Signatures / overloading(오버로딩) (0) | 2022.05.10 |
[TS]03 unKnown / void / never (0) | 2022.05.10 |
댓글